Table of Contents
Scrolling to a Specific Area
If you need to scroll the web viewer to a specific area on the screen, you can use JavaScript.
Syntax: window.scrollTo( xpos, ypos )
Parameter Type Description
xpos | Number | Required. The coordinate to scroll to, along the x-axis (horizontal), in pixels |
ypos | Number | Required. The coordinate to scroll to, along the y-axis (vertical), in pixels |
In the following Scripting Engine code, we scroll to position 300px down and 100px right.
runScript("""window.scrollTo(100,300)""")
Scrolling by Specific Pixels
If you need to scroll the web viewer by specific pixels on the screen, you can use JavaScript.
Syntax: window.scrollBy( xnum, ynum )
Parameter Type Description
xnum | Number | Required. How many pixels to scroll by along the x-axis (horizontal). Positive values will scroll to the right, while negative values will scroll to the left |
ynum | Number | Required. How many pixels to scroll by along the y-axis (vertical). Positive values will scroll down, while negative values scroll up. |
In the following Scripting Engine code, we scroll by position 300px down and 100px right.
runScript("""window.scrollBy(100,300)""")
How to find how many pixels to scroll
You could likely do it on your PC. Open up the page you want to show in Chrome (or Edge) on your PC and hit F12. That should show Dev Tools (other browsers have similar stuff). Scroll the page to where you want it, and then in the “Console” section of the Dev Tools, type:
window.scrollY
That should show you how many pixels you have actually scrolled down. And you can use this value.
Deleting a Specific Element
runScript("""j("css_selector").remove();""")
Moving an Element to Body
runScript("""var elem = j("css_selector");j("body").append(elem);""")
Delete All Content Besides One Element
extract("""css_selector""")
Change the Background Color
runScript("""document.body.style.backgroundColor = "white";""")
Or you can use a hex color picker:
runScript("""document.body.style.backgroundColor = "#6217e5";""")
The #6217e5 is this color:
Selecting a Specific Option from a Dropdown List
runScript(""" a = document.querySelector('Dropdown_list's_CSS_Selector') for (var i = 0; i < a.options.length; i++) { if (a.options[i].text === '_DROPDOWN_VALUE_') { a.selectedIndex = i; break; } } """)
-
_DROPDOWN_VALUE_ should be an option from the dropdown menu
Inject any CSS code
runScript("""var css = 'div{color: red} header{display: none !important;} * {font-size: 25px}', head = document.head || document.getElementsByTagName('head')[0], style = document.createElement('style'); head.appendChild(style); style.type = 'text/css'; if (style.styleSheet){ // This is required for IE8 and below. style.styleSheet.cssText = css; } else { style.appendChild(document.createTextNode(css)); }""")
Example web page before applying the above script code:
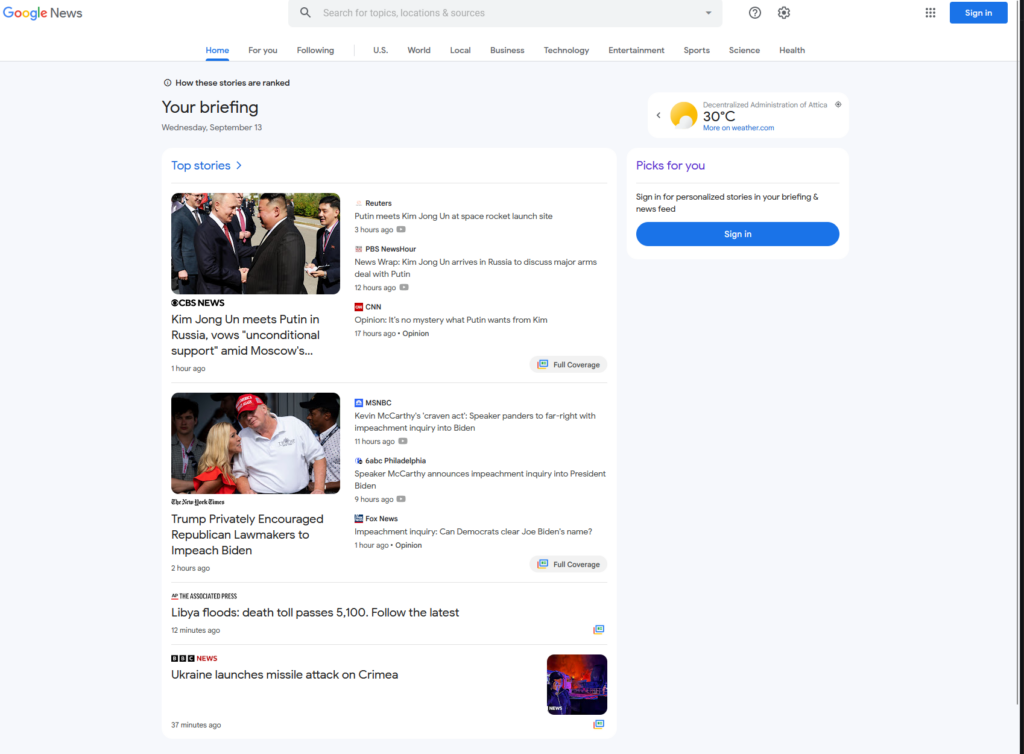
Example web page after applying the above script code:
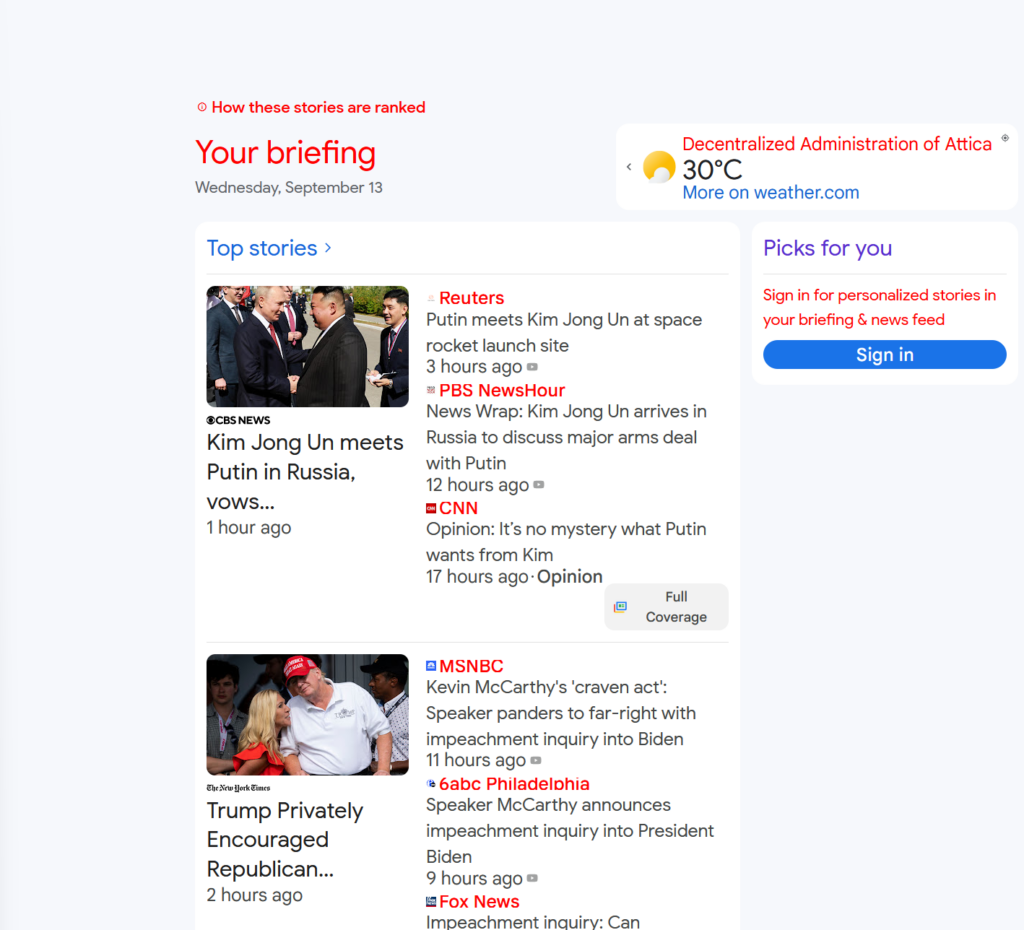